Zip Component
Overview
The Zip component provides industry-standard Zip archive functionality. It is designed to be easy to use. You can pack/unpack a file or folder with a single line of code. If you need to create or extract Zip files on the fly, this component is for you. This component can be used in environments that support COM such as Active Server Pages, Windows Scripting Host, Visual Basic, etc.
- License
- Freeware
- Type
- 32-bit ActiveX DLL
- Version
- 2.5
- File Name
- XZip.dll
- Download Package
- x-zip.zip
Download
Download Zip Component
Visual C++ users can download header files.
Installation Instructions
- Move the dll to a directory like: C:\Program Files\XStandard\Bin\.
- Open a command prompt and
cd
to the directory where the dll is located. - Type regsvr32 XZip.dll or on 64-bit OS type C:\Windows\SysWOW64\regsvr32.exe XZip.dll
- Grant "Read & Execute" file permissions on this dll to Everyone.
Note, the command prompt must be "Run as administrator" as shown in the screen shot below.
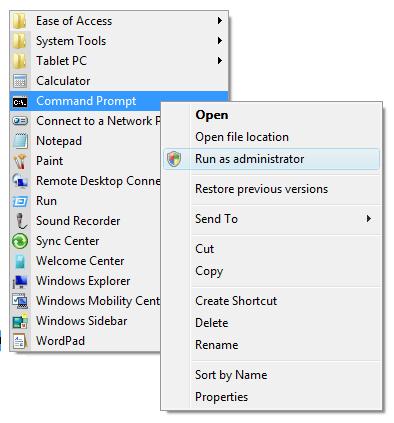
Uninstall Instructions
- Open a command prompt and
cd
to the directory where the dll is located. - Type regsvr32 -u XZip.dll or on 64-bit OS type C:\Windows\SysWOW64\regsvr32.exe -u XZip.dll
Usage from 64-bit OS
Please see instructions for 64-bit OS usage.
API Reference: ProgID: XStandard.Zip
Sub Pack(sFilePath As String, sArchive As String, [bStorePath As Boolean = False], [sNewPath As String], [lCompressionLevel As Long = -1])
Add file or folder to an archive. Compression level 1 is minimum, level 9 is maximum, all other values default to level 6.
Sub UnPack(sArchive As String, sFolderPath As String, [sPattern As String])
Extract contents of an archive to a folder.
Sub Delete(sFile As String, sArchive As String)
Remove a file from an archive.
Sub Move(sFrom As String, sTo As String, sArchive As String)
Move or rename a file in the archive.
Function Contents(sArchive As String) As Items
Get a list of files and folder in the archive.
Property ErrorCode As Long
(read-only)
Return the code of last operation. The following are possible error codes:
Error code | Description |
---|
200 | Archive file is not correct. |
201 | Archive file is not a valid zip file[header]. |
202 | Archive file is not a valid zip file[dir]. |
203 | Cannot create archive file. |
204 | Compressed header is not correct |
205 | File size is not correct. |
206 | Cannot Alloc memory |
207 | Cannot open archive file |
208 | Archive file is empty |
209 | Cannot alloc memeory. |
210 | Cannot find source file. |
211 | Cannot open file |
212 | Cannot alloc memory. |
213 | Cannot alloc memory. |
214 | There is no file. |
215 | Archive file is same as the input file. |
216 | Cannot Alloc memory. |
217 | Incorrect signature of header |
230 | Cannot open archive file. |
240 | Archive file is not correct. |
241 | Archive file is not a valid zip file[header]. |
242 | Archive file is not a valid zip file[dir]. |
250 | Cannot open archive file. |
251 | Archive file is not correct. |
252 | Cannot create file for swapping. |
253 | Header of archive file is incorrect. |
254 | Unknown error when modifying archive file. |
290 | Cannot get information from archive file. |
291 | Cannot get information from archive file. |
591 | Cannot get information from archive file. |
Property ErrorDescription As String
(read-only)
Return the error code description of last operation.
Property Version As String
(read-only)
Product version.
API Reference: Class: Items
Property Count As Long
(read-only)
Returns the number of members in a collection.
Property Item(Index As Long) As Item
(read-only)
Returns a specific member of a collection by position.
API Reference: Class: Item
Property Date As Date
(read-only)
Last modified date.
Property Name As String
(read-only)
File name.
Property Path As String
(read-only)
Relative path.
Property Size As Long
(read-only)
File size in bytes.
Property Type As ItemType
(read-only)
Type of object.
API Reference: Enum: ItemType
Const tFolder = 1
Item is a folder.
Const tFile = 2
Item is a file.
Examples
The examples below are for Active Server Pages. For Windows Scripting Host or Visual Basic, replace Server.CreateObject
with CreateObject
and replace Resonse.Write
with MsgBox
.
How to archive (or zip) multiple files
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Pack "C:\Temp\golf.jpg", "C:\Temp\images.zip"
objZip.Pack "C:\Temp\racing.gif", "C:\Temp\images.zip"
Set objZip = Nothing
%>
How to archive (or zip) multiple files with different compression levels
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Pack "C:\Temp\reports.doc", "C:\Temp\archive.zip", , , 9
objZip.Pack "C:\Temp\boat.jpg", "C:\Temp\archive.zip", , , 1
Set objZip = Nothing
%>
How to archive (or zip) multiple files with default path
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Pack "C:\Temp\reports.doc", "C:\Temp\archive.zip", True
objZip.Pack "C:\Temp\boat.jpg", "C:\Temp\archive.zip", True
Set objZip = Nothing
%>
How to archive (or zip) multiple files with a custom path
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Pack "C:\Temp\reports.doc", "C:\Temp\archive.zip", True, "files/word"
objZip.Pack "C:\Temp\boat.jpg", "C:\Temp\archive.zip", True, "files/images"
Set objZip = Nothing
%>
How to archive (or zip) multiple files using wildcards
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Pack "C:\Temp\*.jpg", "C:\Temp\images.zip"
Set objZip = Nothing
%>
How to unzip files
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.UnPack "C:\Temp\images.zip", "C:\Temp\"
Set objZip = Nothing
%>
How to unzip files using wildcards
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.UnPack "C:\Temp\images.zip", "C:\Temp\", "*.jpg"
Set objZip = Nothing
%>
How to get a listing of files and folder in an archive
<%
Dim objZip, objItem
Set objZip = Server.CreateObject("XStandard.Zip")
For Each objItem In objZip.Contents("C:\Temp\images.zip")
Response.Write objItem.Path & objItem.Name & "<br />"
Next
Set objZip = Nothing
Set objItem = Nothing
%>
How to remove a file from an archive
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Delete "headshots/smith.jpg", "C:\Temp\images.zip"
Set objZip = Nothing
%>
How to move a file in an archive
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Move "headshots/jones.jpg", "staff/jones.jpg", "C:\Temp\images.zip"
Set objZip = Nothing
%>
How to rename a file in an archive
<%
Dim objZip
Set objZip = Server.CreateObject("XStandard.Zip")
objZip.Move "headshots/jones.jpg", "headshots/randy-jones.jpg", "C:\Temp\images.zip"
Set objZip = Nothing
%>
Known Issues
- The
UnPack()
method will first remove all files from the destination folder. This behavior will change in future releases. - This component was not designed to work with huge archives. Max archive size depends on the amount of available RAM.
- This component is designed for 32-bit operating systems and will not work natively on 64-bit operating systems.